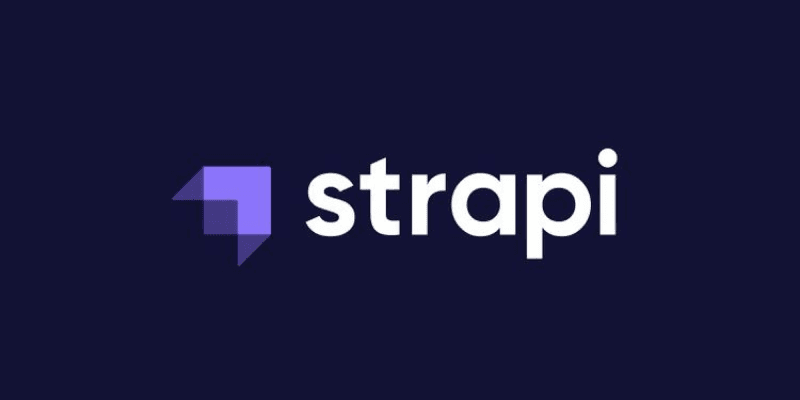
Recently I have adopted Strapi as my favourite headless CMS solution.
I love the fact it is free, open source and completely customisable although it is fair to say that those things I love about Strapi make it a somewhat steep learning curve in comparison to a strictly commercial enterprise such as Contentful.
With Contentful, it is essentially a case of learning their API and getting to grips with the the GUI (graphical user interface) and then you are good to go!
When you first get started with Strapi, along with learning the API and getting to grips with the GUI, you have to consider many more things such as choosing a database, connecting to the database, different environment configurations, where to host your Strapi CMS, configuring plugins and many more things.
However, in my opinion it is more than worth it spending some time to understand how it all works as ultimately the benefits such as avoiding vendor lock-in and total customisation make it a much more viable and cheaper option going forwards.
Strapi + Sendinblue + Next.js
One area I have been investigating recently is Strapi custom routes and controllers.
I usually use Sendinblue as my preferred CRM for my projects and I needed to figure out how to configure Strapi to add newsletter subscriber contacts to my email list at Sendinblue when a new person hits the ‘subscribe’ button from a Next.js frontend.
I have detailed below the process required to be able to complete this.
N.B I have assumed a basic understanding of Strapi, Next.js and Sendinblue
Firstly, I realised that I did not need to create a new content model from the Strapi dashboard because I am not storing any data directly in Strapi from these custom routes and controllers. Instead, you only need to work directly in our backend files.
In the api folder, create a new
contact
folder (you can call it anything, I just decided to call it contact)mkdir /api/contact
Inside the
contact
folder, create two foldersmkdir /api/contact/config
andmkdir /api/contact/controllers
Inside
/api/contact/config
create a new json filetouch /api/contact/config/routes.json
Define a new route with the path
/contacts/newsletter
with the handler asContact.newsletter
. This is an important bit to remember as the controller will use the name as provided by handler i.e "newsletter".{ "routes": [ { "method": "POST", "path": "/contacts/newsletter", "handler": "Contact.newsletter", "config": { "policies": [] } } ] }
In the
/api/contact/controllers
folder, create a new filetouch /api/contact/controllers/contact.js
Inside the
/contact.js
file we need to define our controllers. As we used the “handler” of “Contact.newsletter”, we will create the “newsletter” controller like so:module.exports = { async newsletter(ctx) { } }
This controller is now hooked into the route so when we fetch
POST /contacts/newsletter
it triggers this function.Next, we need to implement the logic to add the newsletter subscriber to my Sendinblue list. For this we will use the Sendinblue Node API library
npm install sib-api-v3-sdk
Once installed, add the following to
/api/contact/controllers/contact/js
. Please note that you will need to create a API key in Sendinblue and then store this in in yourStrapi /.env
file as something likeSIB_API_KEY
const SibApiV3Sdk = require('sib-api-v3-sdk'); const defaultClient = SibApiV3Sdk.ApiClient.instance; let apiKey = defaultClient.authentications['api-key']; apiKey.apiKey = process.env.SIB_API_KEY;
I then created a separate function to handle adding the contact to a list which takes the email and an array of Sendinblue lists which we want to add the contact to:
const createNewContact = async ({ email, listIds }) => { const apiInstance = new SibApiV3Sdk.ContactsApi(); let createContact = new SibApiV3Sdk.CreateContact(); createContact.email = email; createContact.listIds = listIds; try { const res = await apiInstance.createContact(createContact); return res; } catch (err) { const { status } = err.response.error; return status; } }
Now we just need to call the
createNewContact()
function from within our controller to complete the custom controller. Note that listIds: [18] just indicates that I am adding new subscribers to Sendinblue list 18.module.exports = { async newsletter(ctx) { const { email } = ctx.request.body; const result = await createNewContact({ email, listIds: [18], }); if (result === 400) return ctx.send({ status: 400, msg: 'You have already subscribed to our newsletter.', }); return ctx.send({ status: 201, msg: 'Thank you for subscribing to my newsletter.', }); } }
Now we can call this from our Next.js frontend
export const addNewsletter = async (email) => { try { const url = `${process.env.NEXT_PUBLIC_STRAPI_API_URL}/contacts/newsletter` const res = await axios.post(url, { email, }); return res.data.msg; } catch (error) { return error; } };
If you followed this guide correctly, then by calling POST
/[YOUR_STRAPI_CMS]/contacts/newsletter
you'll be able to add contacts to Sendinblue via your Strapi CSM.One final point to note is that you will need to go to your Strapi dashboard and enable
contact
in settings>roles>public otherwise you will get a 400 unauthorized error.